Add a Snackbar
suggest changeOne of the main features in Material Design is the addition of a Snackbar
, which in theory replaces the previous Toast
. As per the Android documentation:
Snackbars contain a single line of text directly related to the operation performed. They may contain a text action, but no icons. Toasts are primarily used for system messaging. They also display at the bottom of the screen, but may not be swiped off-screen.
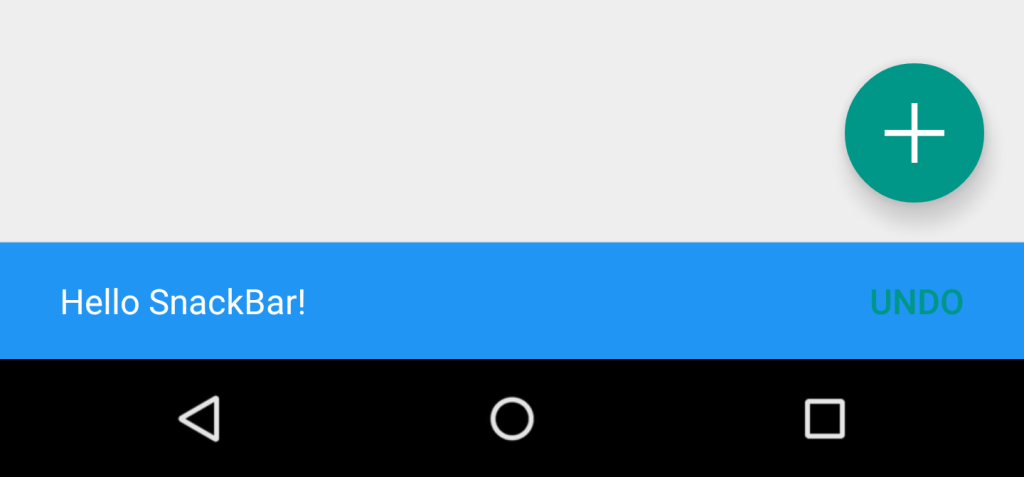
Toasts can still be used in Android to display messages to users, however if you have decided to opt for material design usage in your app, it is recommended that you actually use a snackbar. Instead of being displayed as an overlay on your screen, a Snackbar
pops from the bottom.
Here is how it is done:
Snackbar snackbar = Snackbar
.make(coordinatorLayout, "Here is your new Snackbar", Snackbar.LENGTH_LONG);
snackbar.show();
As for the length of time to show the Snackbar
, we have the options similar to the ones offered by a Toast
or we could set a custom duration in milliseconds:
LENGTH_SHORT
LENGTH_LONG
LENGTH_INDEFINITE
setDuration()
(since version22.2.1
)
You can also add dynamic features to your Snackbar
such as ActionCallback
or custom color. However do pay attention to the design guideline offered by Android when customising a Snackbar
.
Implementing the Snackbar
has one limitation however. The parent layout of the view you are going to implement a Snackbar
in needs to be a CoordinatorLayout
. This is so that the actual popup from the bottom can be made.
This is how to define a CoordinatorLayout
in your layout xml file:
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/coordinatorLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
//any other widgets in your layout go here.
</android.support.design.widget.CoordinatorLayout>
The CoordinatorLayout
then needs to be defined in your Activity’s onCreate
method, and then used when creating the Snackbar
itself.
For more information about about the Snackbar
, please check the official documentation or the dedicated topic in the documentation.