Common memory leaks and how to fix them
suggest change1. Fix your contexts:
Try using the appropriate context: For example since a Toast can be seen in many activities instead of in just one, use getApplicationContext()
for toasts, and since services can keep running even though an activity has ended start a service with:
Intent myService = new Intent(getApplicationContext(), MyService.class);
Use this table as a quick guide for what context is appropriate:
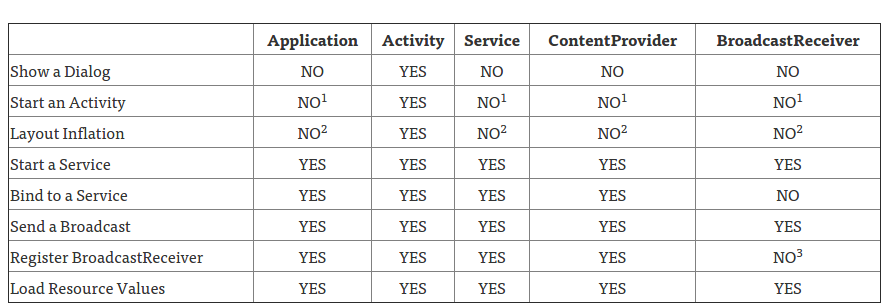
Original article on context here.
2. Static reference to Context
A serious memory leak mistake is keeping a static reference to View
. Every View
has an inner reference to the Context
. Which means an old Activity with its whole view hierarchy will not be garbage collected until the app is terminated. You will have your app twice in memory when rotating the screen.
Make sure there is absolutely no static reference to View, Context or any of their descendants.
3. Check that you’re actually finishing your services.
For example, I have an intentService that uses the Google location service API. And I forgot to call googleApiClient.disconnect();
:
//Disconnect from API onDestroy()
if (googleApiClient.isConnected()) {
LocationServices.FusedLocationApi.removeLocationUpdates(googleApiClient, GoogleLocationService.this);
googleApiClient.disconnect();
}
4. Check image and bitmaps usage:
If you are using Square’s library Picasso I found I was leaking memory by not using the .fit()
, that drastically reduced my memory footprint from 50MB in average to less than 19MB:
Picasso.with(ActivityExample.this) //Activity context
.load(object.getImageUrl())
.fit() //This avoided the OutOfMemoryError
.centerCrop() //makes image to not stretch
.into(imageView);
5. If you are using broadcast receivers unregister them.
6. If you are using java.util.Observer
(Observer pattern):
Make sure to use deleteObserver(observer);