Creating Custom Views
suggest changeIf you need a completely customized view, you’ll need to subclass View
(the superclass of all Android views) and provide your custom sizing (onMeasure(...)
) and drawing (onDraw(...)
) methods:
- Create your custom view skeleton: this is basically the same for every custom view. Here we create the skeleton for a custom view that can draw a smiley, called
SmileyView
:
public class SmileyView extends View {
private Paint mCirclePaint;
private Paint mEyeAndMouthPaint;
private float mCenterX;
private float mCenterY;
private float mRadius;
private RectF mArcBounds = new RectF();
public SmileyView(Context context) {
this(context, null, 0);
}
public SmileyView(Context context, AttributeSet attrs) {
this(context, attrs, 0);
}
public SmileyView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
initPaints();
}
private void initPaints() {/* ... */}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {/* ... */}
@Override
protected void onDraw(Canvas canvas) {/* ... */}
}
- Initialize your paints: the
Paint
objects are the brushes of your virtual canvas defining how your geometric objects are rendered (e.g. color, fill and stroke style, etc.). Here we create twoPaint
s, one yellow filled paint for the circle and one black stroke paint for the eyes and the mouth:
private void initPaints() {
mCirclePaint = new Paint(Paint.ANTI_ALIAS_FLAG);
mCirclePaint.setStyle(Paint.Style.FILL);
mCirclePaint.setColor(Color.YELLOW);
mEyeAndMouthPaint = new Paint(Paint.ANTI_ALIAS_FLAG);
mEyeAndMouthPaint.setStyle(Paint.Style.STROKE);
mEyeAndMouthPaint.setStrokeWidth(16 * getResources().getDisplayMetrics().density);
mEyeAndMouthPaint.setStrokeCap(Paint.Cap.ROUND);
mEyeAndMouthPaint.setColor(Color.BLACK);
}
- Implement your own
onMeasure(...)
method: this is required so that the parent layouts (e.g.FrameLayout
) can properly align your custom view. It provides a set ofmeasureSpecs
that you can use to determine your view’s height and width. Here we create a square by making sure that the height and width are the same:
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
int w = MeasureSpec.getSize(widthMeasureSpec);
int h = MeasureSpec.getSize(heightMeasureSpec);
int size = Math.min(w, h);
setMeasuredDimension(size, size);
}
Note that onMeasure(...)
must contain at least one call to setMeasuredDimension(..)
or else your custom view will crash with an IllegalStateException
.
- Implement your own
onSizeChanged(...)
method: this allows you to catch the current height and width of your custom view to properly adjust your rendering code. Here we just calculate our center and our radius:
@Override
protected void onSizeChanged(int w, int h, int oldw, int oldh) {
mCenterX = w / 2f;
mCenterY = h / 2f;
mRadius = Math.min(w, h) / 2f;
}
- Implement your own
onDraw(...)
method: this is where you implement the actual rendering of your view. It provides aCanvas
object that you can draw on (see the officialCanvas
documentation for all drawing methods available).
@Override
protected void onDraw(Canvas canvas) {
// draw face
canvas.drawCircle(mCenterX, mCenterY, mRadius, mCirclePaint);
// draw eyes
float eyeRadius = mRadius / 5f;
float eyeOffsetX = mRadius / 3f;
float eyeOffsetY = mRadius / 3f;
canvas.drawCircle(mCenterX - eyeOffsetX, mCenterY - eyeOffsetY, eyeRadius, mEyeAndMouthPaint);
canvas.drawCircle(mCenterX + eyeOffsetX, mCenterY - eyeOffsetY, eyeRadius, mEyeAndMouthPaint);
// draw mouth
float mouthInset = mRadius /3f;
mArcBounds.set(mouthInset, mouthInset, mRadius * 2 - mouthInset, mRadius * 2 - mouthInset);
canvas.drawArc(mArcBounds, 45f, 90f, false, mEyeAndMouthPaint);
}
- Add your custom view to a layout: the custom view can now be included in any layout files that you have. Here we just wrap it inside a
FrameLayout
:
<FrameLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.example.app.SmileyView
android:layout_width="match_parent"
android:layout_height="match_parent" />
</FrameLayout>
Note that it is recommended to build your project after the view code is finished. Without building it you won’t be able to see the view on a preview screen in Android Studio.
After putting everything together, you should be greeted with the following screen after launching the activity containing the above layout:
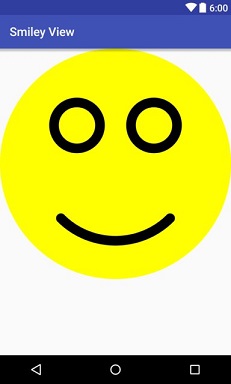
Found a mistake? Have a question or improvement idea?
Let me know.
Table Of Contents