Custom Alert Dialog with EditText
suggest changevoid alertDialogDemo() {
// get alert_dialog.xml view
LayoutInflater li = LayoutInflater.from(getApplicationContext());
View promptsView = li.inflate(R.layout.alert_dialog, null);
AlertDialog.Builder alertDialogBuilder = new AlertDialog.Builder(
getApplicationContext());
// set alert_dialog.xml to alertdialog builder
alertDialogBuilder.setView(promptsView);
final EditText userInput = (EditText) promptsView.findViewById(R.id.etUserInput);
// set dialog message
alertDialogBuilder
.setCancelable(false)
.setPositiveButton("OK", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
// get user input and set it to result
// edit text
Toast.makeText(getApplicationContext(), "Entered: "+userInput.getText().toString(), Toast.LENGTH_LONG).show();
}
})
.setNegativeButton("Cancel",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
dialog.cancel();
}
});
// create alert dialog
AlertDialog alertDialog = alertDialogBuilder.create();
// show it
alertDialog.show();
}
Xml file: res/layout/alert_dialog.xml
<LinearLayout xmlns:android=“http://schemas.android.com/apk/res/android”
android:id="@+id/layout_root"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical"
android:padding="10dp" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Type Your Message : "
android:textAppearance="?android:attr/textAppearanceLarge" />
<EditText
android:id="@+id/etUserInput"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
<requestFocus />
</EditText>
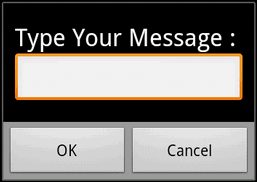
Found a mistake? Have a question or improvement idea?
Let me know.
Table Of Contents