Custom Google Map Styles
suggest changeMap Style
Google Maps come with a set of different styles to be applied, using this code :
// Sets the map type to be "hybrid"
map.setMapType(GoogleMap.MAP_TYPE_HYBRID);
The different map styles are :
Normal
map.setMapType(GoogleMap.MAP_TYPE_NORMAL);
Typical road map. Roads, some man-made features, and important natural features such as rivers are shown. Road and feature labels are also visible.
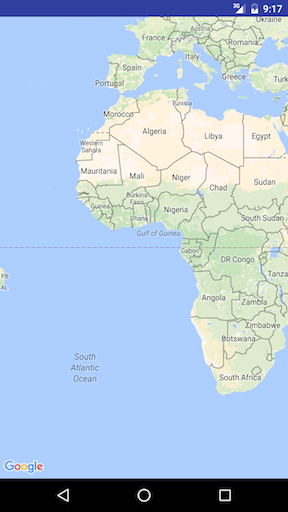
Hybrid
map.setMapType(GoogleMap.MAP_TYPE_HYBRID);
Satellite photograph data with road maps added. Road and feature labels are also visible.
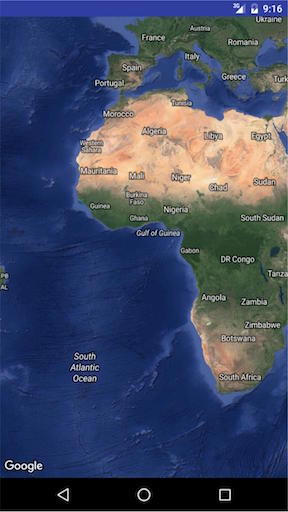
Satellite
map.setMapType(GoogleMap.MAP_TYPE_SATELLITE);
Satellite photograph data. Road and feature labels are not visible.
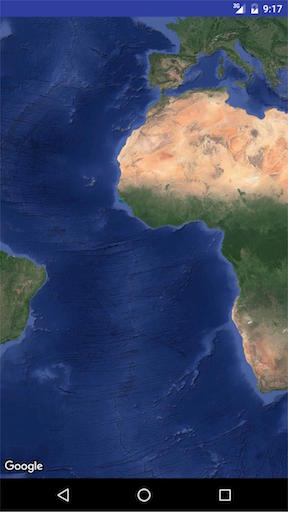
Terrain
map.setMapType(GoogleMap.MAP_TYPE_TERRAIN);
Topographic data. The map includes colors, contour lines and labels, and perspective shading. Some roads and labels are also visible.
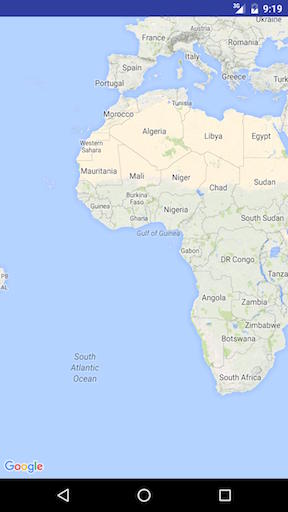
None
map.setMapType(GoogleMap.MAP_TYPE_NONE);
No tiles. The map will be rendered as an empty grid with no tiles loaded.
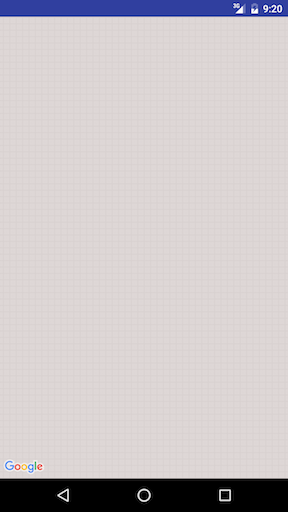
OTHER STYLE OPTIONS
Indoor Maps
At high zoom levels, the map will show floor plans for indoor spaces. These are called indoor maps, and are displayed only for the ‘normal’ and ‘satellite’ map types.
to enable or disable indoor maps, this is how it’s done :
GoogleMap.setIndoorEnabled(true).
GoogleMap.setIndoorEnabled(false).
We can add custom styles to maps.
In onMapReady method add the following code snippet
mMap = googleMap;
try {
// Customise the styling of the base map using a JSON object defined
// in a raw resource file.
boolean success = mMap.setMapStyle(
MapStyleOptions.loadRawResourceStyle(
MapsActivity.this, R.raw.style_json));
if (!success) {
Log.e(TAG, "Style parsing failed.");
}
} catch (Resources.NotFoundException e) {
Log.e(TAG, "Can't find style.", e);
}
under res folder create a folder name raw and add the styles json file. Sample style.json file
[
{
"featureType": "all",
"elementType": "geometry",
"stylers": [
{
"color": "#242f3e"
}
]
},
{
"featureType": "all",
"elementType": "labels.text.stroke",
"stylers": [
{
"lightness": -80
}
]
},
{
"featureType": "administrative",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#746855"
}
]
},
{
"featureType": "administrative.locality",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#d59563"
}
]
},
{
"featureType": "poi",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#d59563"
}
]
},
{
"featureType": "poi.park",
"elementType": "geometry",
"stylers": [
{
"color": "#263c3f"
}
]
},
{
"featureType": "poi.park",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#6b9a76"
}
]
},
{
"featureType": "road",
"elementType": "geometry.fill",
"stylers": [
{
"color": "#2b3544"
}
]
},
{
"featureType": "road",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#9ca5b3"
}
]
},
{
"featureType": "road.arterial",
"elementType": "geometry.fill",
"stylers": [
{
"color": "#38414e"
}
]
},
{
"featureType": "road.arterial",
"elementType": "geometry.stroke",
"stylers": [
{
"color": "#212a37"
}
]
},
{
"featureType": "road.highway",
"elementType": "geometry.fill",
"stylers": [
{
"color": "#746855"
}
]
},
{
"featureType": "road.highway",
"elementType": "geometry.stroke",
"stylers": [
{
"color": "#1f2835"
}
]
},
{
"featureType": "road.highway",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#f3d19c"
}
]
},
{
"featureType": "road.local",
"elementType": "geometry.fill",
"stylers": [
{
"color": "#38414e"
}
]
},
{
"featureType": "road.local",
"elementType": "geometry.stroke",
"stylers": [
{
"color": "#212a37"
}
]
},
{
"featureType": "transit",
"elementType": "geometry",
"stylers": [
{
"color": "#2f3948"
}
]
},
{
"featureType": "transit.station",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#d59563"
}
]
},
{
"featureType": "water",
"elementType": "geometry",
"stylers": [
{
"color": "#17263c"
}
]
},
{
"featureType": "water",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#515c6d"
}
]
},
{
"featureType": "water",
"elementType": "labels.text.stroke",
"stylers": [
{
"lightness": -20
}
]
}
]
To generate styles json file click this link
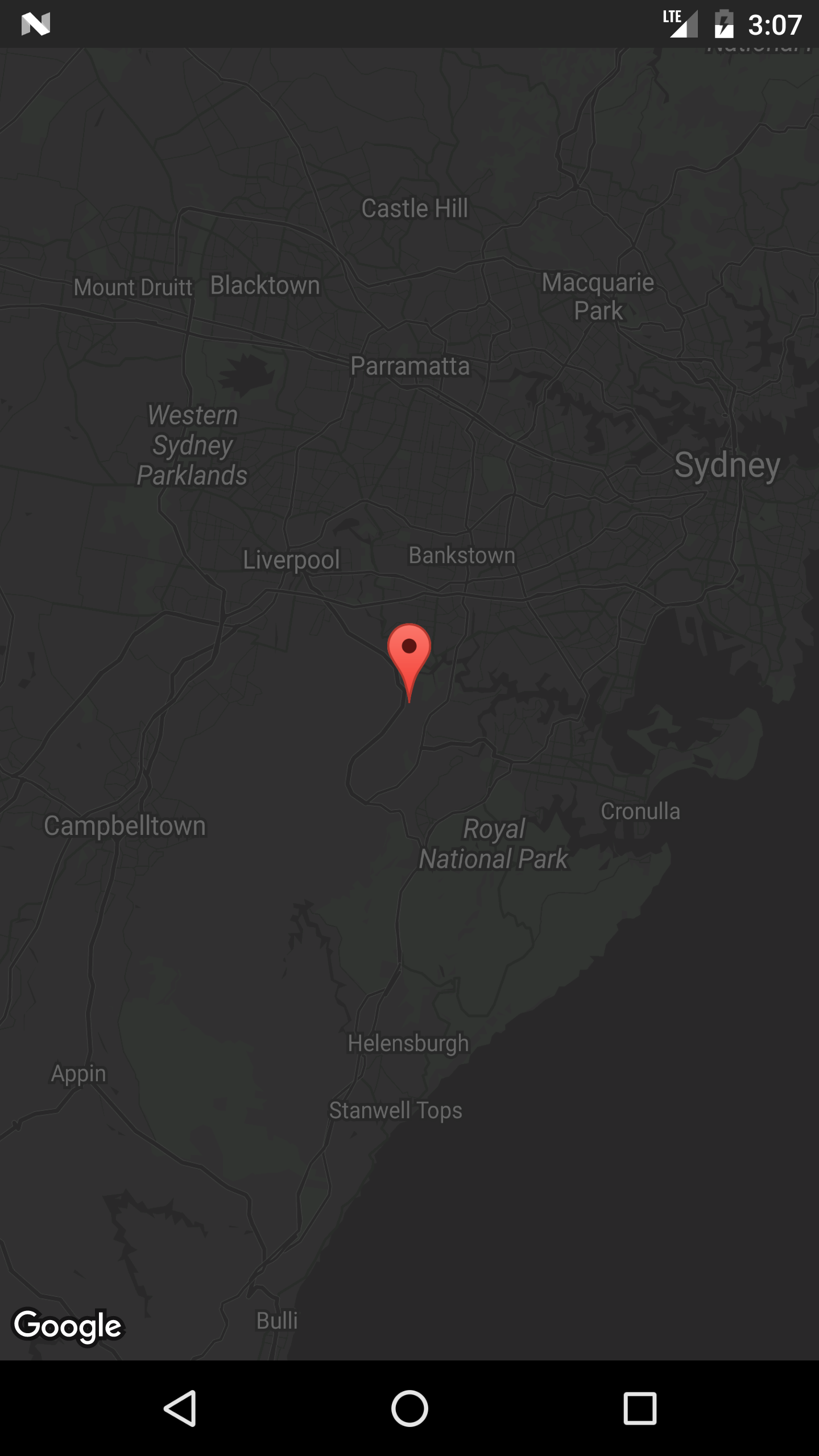