Firebase Realtime Database how to setget data
suggest changeNote: Let’s setup some anonymous authentication for the example
{
"rules": {
".read": "auth != null",
".write": "auth != null"
}
}
Once it is done, create a child by editing your database address. For example:
https://your-project.firebaseio.com/ to https://your-project.firebaseio.com/chat
We will put data to this location from our Android device. You don’t have to create the database structure (tabs, fields… etc), it will be automatically created when you’ll send Java object to Firebase!
Create a Java object that contains all the attributes you want to send to the database:
public class ChatMessage {
private String username;
private String message;
public ChatMessage(String username, String message) {
this.username = username;
this.message = message;
}
public ChatMessage() {} // you MUST have an empty constructor
public String getUsername() {
return username;
}
public String getMessage() {
return message;
}
}
Then in your activity:
if (FirebaseAuth.getInstance().getCurrentUser() == null) {
FirebaseAuth.getInstance().signInAnonymously().addOnCompleteListener(new OnCompleteListener<AuthResult>() {
@Override
public void onComplete(@NonNull Task<AuthResult> task) {
if (task.isComplete() && task.isSuccessful()){
FirebaseDatabase database = FirebaseDatabase.getInstance();
DatabaseReference reference = database.getReference("chat"); // reference is 'chat' because we created the database at /chat
}
}
});
}
To send a value:
ChatMessage msg = new ChatMessage("user1", "Hello World!");
reference.push().setValue(msg);
To receive changes that occurs in the database:
reference.addChildEventListener(new ChildEventListener() {
@Override
public void onChildAdded(DataSnapshot dataSnapshot, String s) {
ChatMessage msg = dataSnapshot.getValue(ChatMessage.class);
Log.d(TAG, msg.getUsername()+" "+msg.getMessage());
}
public void onChildChanged(DataSnapshot dataSnapshot, String s) {}
public void onChildRemoved(DataSnapshot dataSnapshot) {}
public void onChildMoved(DataSnapshot dataSnapshot, String s) {}
public void onCancelled(DatabaseError databaseError) {}
});
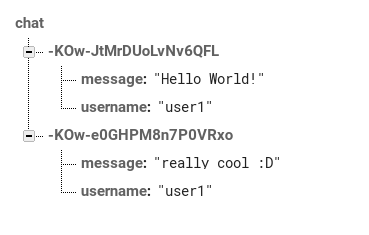
Found a mistake? Have a question or improvement idea?
Let me know.
Table Of Contents