Multiple Themes in one App
suggest changeUsing more than one theme in your Android application, you can add custom colors to every theme, to be like this:
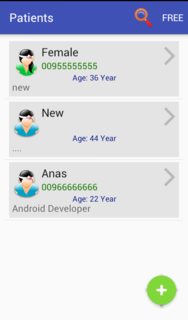
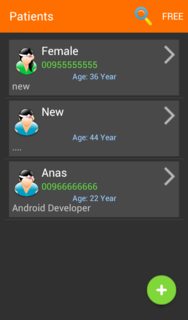
First, we have to add our themes to style.xml
like this:
<style name="OneTheme" parent="Theme.AppCompat.Light.DarkActionBar">
</style>
<!-- -->
<style name="TwoTheme" parent="Theme.AppCompat.Light.DarkActionBar" >
</style>
......
Above you can see OneTheme and TwoTheme.
Now, go to your AndroidManifest.xml
and add this line: android:theme="@style/OneTheme"
to your application tag, this will make OneTheme the default theme:
<application
android:theme="@style/OneTheme"
...>
Create new xml file named attrs.xml
and add this code :
<?xml version="1.0" encoding="utf-8"?>
<resources>
<attr name="custom_red" format="color" />
<attr name="custom_blue" format="color" />
<attr name="custom_green" format="color" />
</resources>
<!-- add all colors you need (just color's name) -->
Go back to style.xml
and add these colors with its values for each theme :
<style name="OneTheme" parent="Theme.AppCompat.Light.DarkActionBar">
<item name="custom_red">#8b030c</item>
<item name="custom_blue">#0f1b8b</item>
<item name="custom_green">#1c7806</item>
</style>
<style name="TwoTheme" parent="Theme.AppCompat.Light.DarkActionBar" >
<item name="custom_red">#ff606b</item>
<item name="custom_blue">#99cfff</item>
<item name="custom_green">#62e642</item>
</style>
Now you have custom colors for each theme, let’s add these color to our views.
Add custom_blue color to the TextView by using “?attr/” :
Go to your imageView and add this color :
<TextView>
android:id="@+id/txte_view"
android:textColor="?attr/custom_blue" />
Mow we can change the theme just by single line setTheme(R.style.TwoTheme);
this line must be before setContentView()
method in onCreate()
method, like this Activity.java
:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setTheme(R.style.TwoTheme);
setContentView(R.layout.main_activity);
....
}
change theme for all activities at once
If we want to change the theme for all activities, we have to create new class named MyActivity extends AppCompatActivity
class (or Activity
class) and add line setTheme(R.style.TwoTheme);
to onCreate() method:
public class MyActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (new MySettings(this).isDarkTheme())
setTheme(R.style.TwoTheme);
}
}
Finally, go to all your activities add make all of them extend the MyActivity base class:
public class MainActivity extends MyActivity {
....
}
In order to change the theme, just go to MyActivity and change R.style.TwoTheme
to your theme (R.style.OneTheme
, R.style.ThreeTheme
….).