Line without blurryness
suggest changeWhen Canvas draws a line it automatically adds anti-aliasing to visually heal “jaggedness”. The result is a line that is less jagged but more blurry.
This function draws a line between 2 points without anti-aliasing using Bresenham’s_line algorithm. The result is a crisp line without the jaggedness.
Important Note: This pixel-by-pixel method is a much slower drawing method than context.lineTo
.
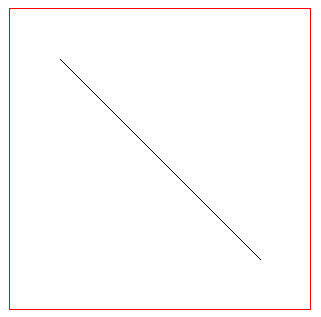
// Usage:
bresenhamLine(50,50,250,250);
// x,y line start
// xx,yy line end
// the pixel at line start and line end are drawn
function bresenhamLine(x, y, xx, yy){
var oldFill = ctx.fillStyle; // save old fill style
ctx.fillStyle = ctx.strokeStyle; // move stroke style to fill
xx = Math.floor(xx);
yy = Math.floor(yy);
x = Math.floor(x);
y = Math.floor(y);
// BRENSENHAM
var dx = Math.abs(xx-x);
var sx = x < xx ? 1 : -1;
var dy = -Math.abs(yy-y);
var sy = y<yy ? 1 : -1;
var err = dx+dy;
var errC; // error value
var end = false;
var x1 = x;
var y1 = y;
while(!end){
ctx.fillRect(x1, y1, 1, 1); // draw each pixel as a rect
if (x1 === xx && y1 === yy) {
end = true;
}else{
errC = 2*err;
if (errC >= dy) {
err += dy;
x1 += sx;
}
if (errC <= dx) {
err += dx;
y1 += sy;
}
}
}
ctx.fillStyle = oldFill; // restore old fill style
}
<!—– //Blindman67 Please note that using while(true) and then break; rather than while(!end) then end = true may seem more effective, Some Javascript optimisers (chrome’s V8) will mark the loop as “indeterminate exit condition” because of the no apparent exit condition “while(true)” and will cause this function and all functions within the script to be marked “Do not optimise” —–!>