How to add a UIImage to a CALayer
suggest changeYou can add an image to a view’s layer
simply by using its contents
property:
myView.layer.contents = UIImage(named: "star")?.CGImage
- Note that the
UIImage
needs to be converted to aCGImage
.
If you wish to add the image in its own layer, you can do it like this:
let myLayer = CALayer()
let myImage = UIImage(named: "star")?.CGImage
myLayer.frame = myView.bounds
myLayer.contents = myImage
myView.layer.addSublayer(myLayer)
Modifying the appearance
The above code produces a view like this. The light blue is the UIView
and the dark blue star is the UIImage
.
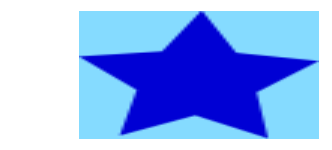
As you can see, though, it looks pixelated. This is because the UIImage
is smaller than the UIView
so it is being scaled to fill the view, which is the default it you don’t specify anything else.
The examples below show variations on the layer’s contentsGravity
property. The code looks like this:
myView.layer.contents = UIImage(named: "star")?.CGImage
myView.layer.contentsGravity = kCAGravityTop
myView.layer.geometryFlipped = true
In iOS, you may want to set the geometryFlipped
property to true
if you are doing anything with top or bottom gravity, otherwise it will be the opposite of what you expect. (Only the gravity is flipped vertically, not the content rendering. If you are having trouble with the content being flipped, see this Stack Overflow answer.)
There are two UIView
examples below for every contentsGravity
setting, one view is larger than the UIImage
and the other is smaller. This way you can see the effects of the scaling and gravity.
kCAGravityResize
This is the default.
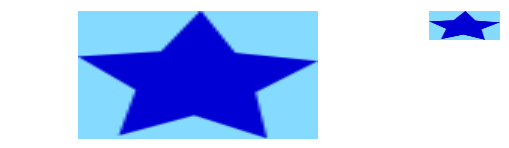
kCAGravityResizeAspect
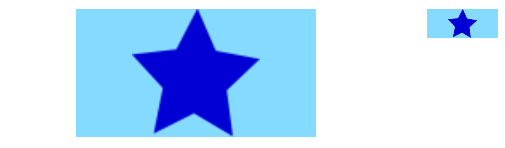
kCAGravityResizeAspectFill
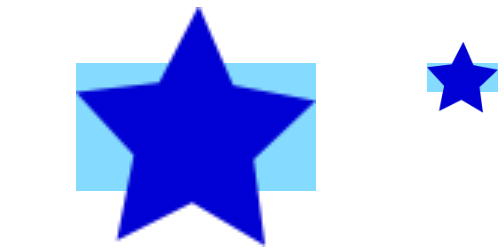
kCAGravityCenter
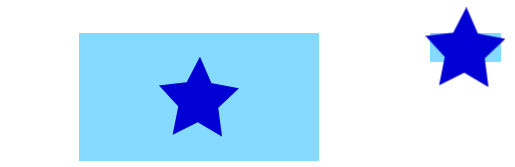
kCAGravityTop
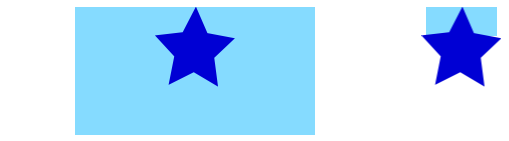
kCAGravityBottom
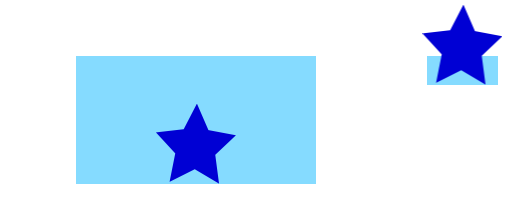
kCAGravityLeft
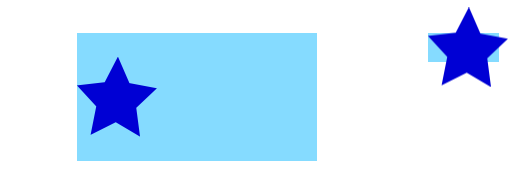
kCAGravityRight
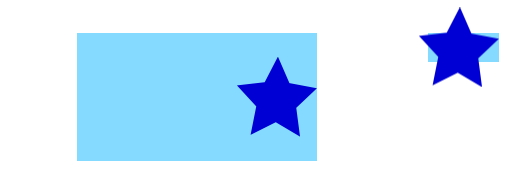
kCAGravityTopLeft
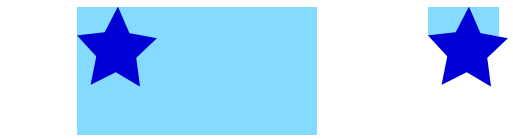
kCAGravityTopRight
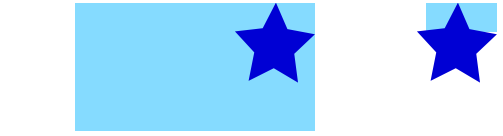
kCAGravityBottomLeft
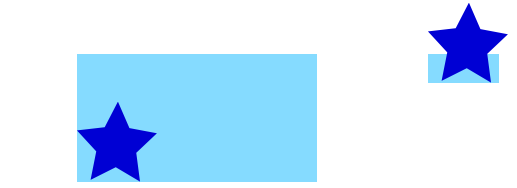
kCAGravityBottomRight
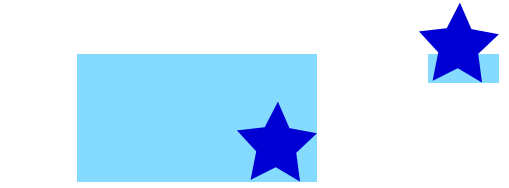
Related
- Content mode property of a view
- Drawing a
UIImage
indrawRect
withCGContextDrawImage
- CALayer Tutorial: Getting Started
Notes
- This example comes originally from this Stack Overflow answer.