Make the view rounded
suggest changeTo make a rounded UIView
, specify a cornerRadius
for the view’s layer
.
This also applies any class which inherits from UIView
, such as UIImageView
.
Programmatically
Swift Code
someImageView.layoutIfNeeded()
someImageView.clipsToBounds = true
someImageView.layer.cornerRadius = 10
Objective-C Code
[someImageView layoutIfNeeded];
someImageView.clipsToBounds = YES;
someImageView.layer.cornerRadius = 10;
Example
//Swift code
topImageView.layoutIfNeeded()
bottomImageView.layoutIfNeeded()
topImageView.clipsToBounds = true
topImageView.layer.cornerRadius = 10
bottomImageView.clipsToBounds = true
bottomImageView.layer.cornerRadius = bottomImageView.frame.width / 2
//Objective-C code
[topImageView layoutIfNeeded]
[bottomImageView layoutIfNeeded];
topImageView.clipsToBounds = YES;
topImageView.layer.cornerRadius = 10;
bottomImageView.clipsToBounds = YES;
bottomImageView.cornerRadius = CGRectGetWidth(bottomImageView.frame) / 2;
Here is the result, showing the rounded view effect using the specified corner radius:
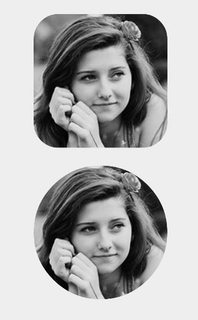
Example
Note
To do this you need to include the QuartzCore framework.
#import <QuartzCore/QuartzCore.h>
Storyboard Configuration
A rounded view effect can also be achieved non-programmatically
by setting the corresponding properties in Storyboard.
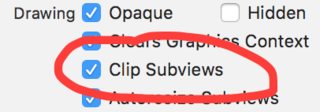
Since layer
properties aren’t exposed in Storyboard, you have to modify the cornerRadius
attribute via the User Defined Runtime Attributes section.
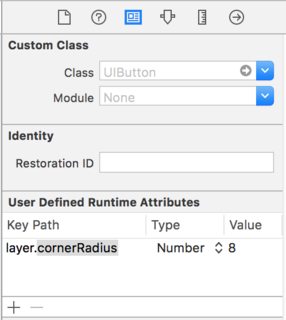
Swift Extension
You can use this handy extension to apply rounded view as long as it has same width and height.
extension UIView {
@discardableResult
public func setAsCircle() -> Self {
self.clipsToBounds = true
let frameSize = self.frame.size
self.layer.cornerRadius = min(frameSize.width, frameSize.height) / 2.0
return self
}
}
To use it:
yourView.setAsCircle()
Found a mistake? Have a question or improvement idea?
Let me know.
Table Of Contents