Request Permission to Use Location Services
suggest changeCheck the app’s authorization status with:
//Swift
let status: CLAuthorizationStatus = CLLocationManager.authorizationStatus()
//Objective-C
CLAuthorizationStatus status = [CLLocationManager authorizationStatus];
Test the status against the follow constants:
//Swift
switch status {
case .NotDetermined:
// Do stuff
case .AuthorizedAlways:
// Do stuff
case .AuthorizedWhenInUse:
// Do stuff
case .Restricted:
// Do stuff
case .Denied:
// Do stuff
}
//Objective-C
switch (status) {
case kCLAuthorizationStatusNotDetermined:
//The user hasn't yet chosen whether your app can use location services or not.
break;
case kCLAuthorizationStatusAuthorizedAlways:
//The user has let your app use location services all the time, even if the app is in the background.
break;
case kCLAuthorizationStatusAuthorizedWhenInUse:
//The user has let your app use location services only when the app is in the foreground.
break;
case kCLAuthorizationStatusRestricted:
//The user can't choose whether or not your app can use location services or not, this could be due to parental controls for example.
break;
case kCLAuthorizationStatusDenied:
//The user has chosen to not let your app use location services.
break;
default:
break;
}
Getting Location Service Permission While App is in Use
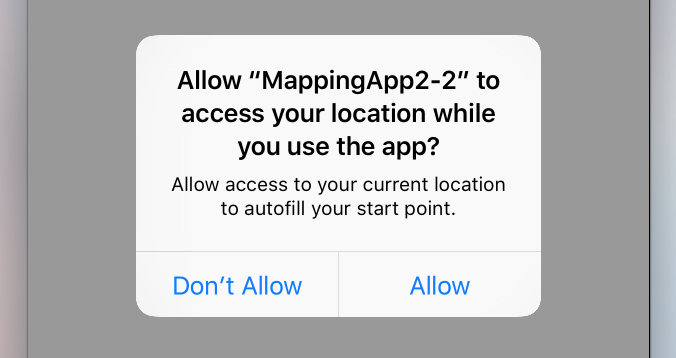
Simplest method is to initialize the location manager as a property of your root view controller and place the permission request in its viewDidLoad
.
This brings up the alert controller that asks for permission:
//Swift
let locationManager = CLLocationManager()
locationManager.requestWhenInUseAuthorization()
//Objective-C
CLLocationManager *locationManager = [[CLLocationManager alloc] init];
[locationManager requestWhenInUseAuthorization];
Add the NSLocationWhenInUseUsageDescription key to your Info.plist. The value will be used in the alert controller’s message
label.
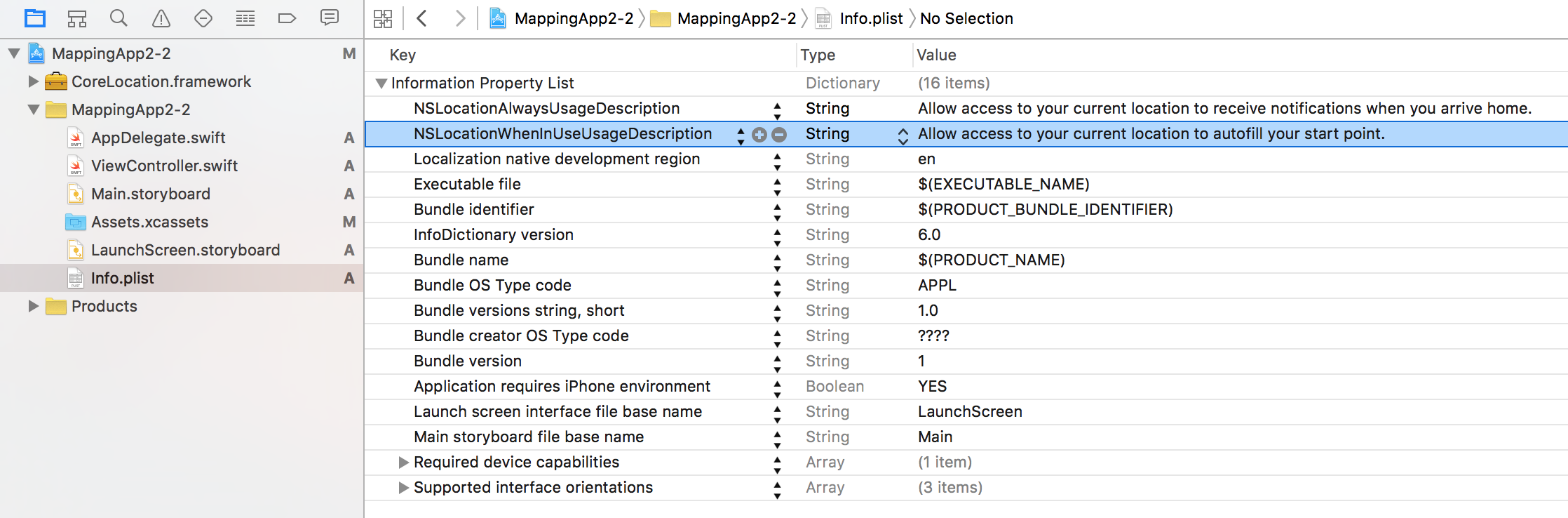
Getting Location Service Permission Always
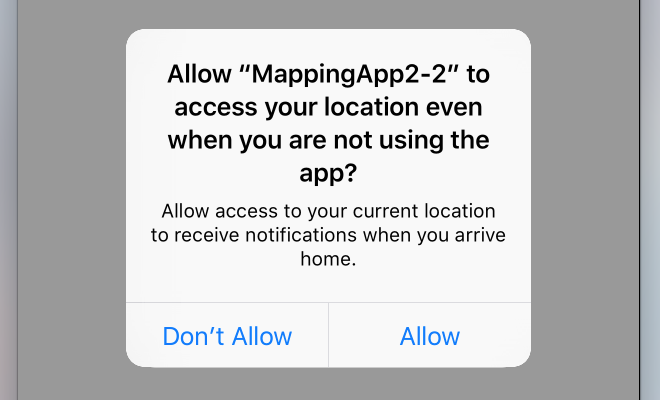
To ask for permission to use location services even when the app is not active, use the following call instead:
//Swift
locationManager.requestAlwaysAuthorization()
//Objective-C
[locationManager requestAlwaysAuthorization];
Then add the NSLocationAlwaysUsageDescription key to your Info.plist. Again, the value will be used in the alert controller’s message
label.
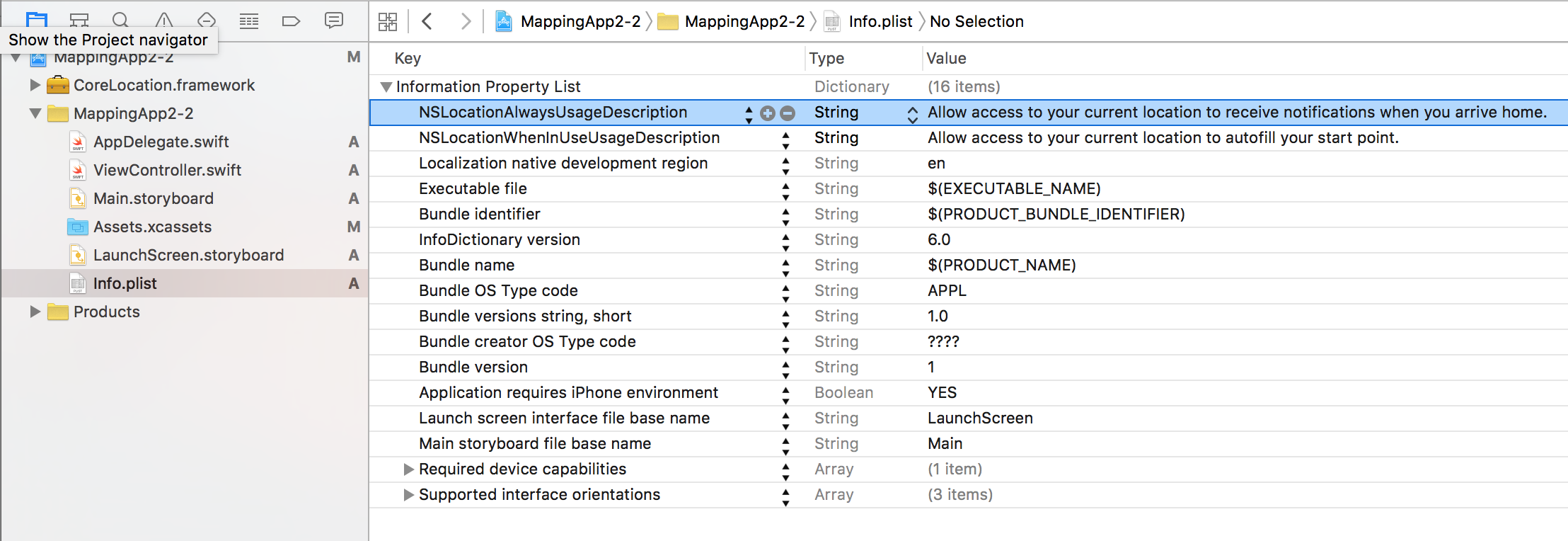
Found a mistake? Have a question or improvement idea?
Let me know.
Table Of Contents