Setup iOS Application Enabling Universal Links
suggest changeThe setup on the app side requires two things:
- Configuring the app’s entitlement, and enabling the universal links by turning on the Associated Domains capability in the project.
- Handling Incoming Links in your
AppDelegate
.
1. Configuring the app’s entitlement, and enabling universal links.
The first step in configuring your app’s entitlements is to enable it for your App ID. Do this in the Apple Developer Member Center. Click on Certificates, Identifiers & Profiles and then Identifiers. Select your App ID (create it first if required), click Edit and enable the Associated Domains entitlement.
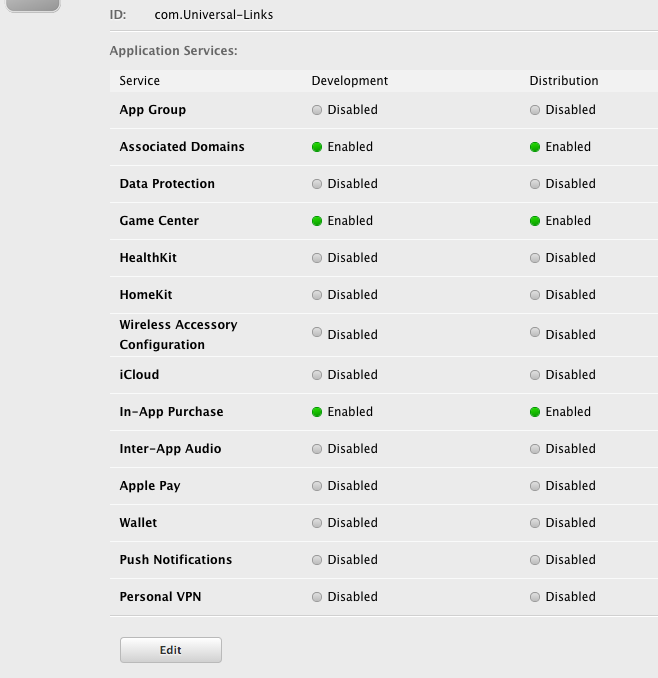
Next, get the App ID prefix and suffix by clicking on the respective App ID.
The App ID prefix and suffix should match the one in the apple-app-site-association file.
Next in Xcode
, select your App’s target, click Capabilities and toggle Associated Domains to On. Add an entry for each domain that your app supports, prefixed with app links:
For example applinks:YourCustomDomainName.com
Which looks like this for the sample app:
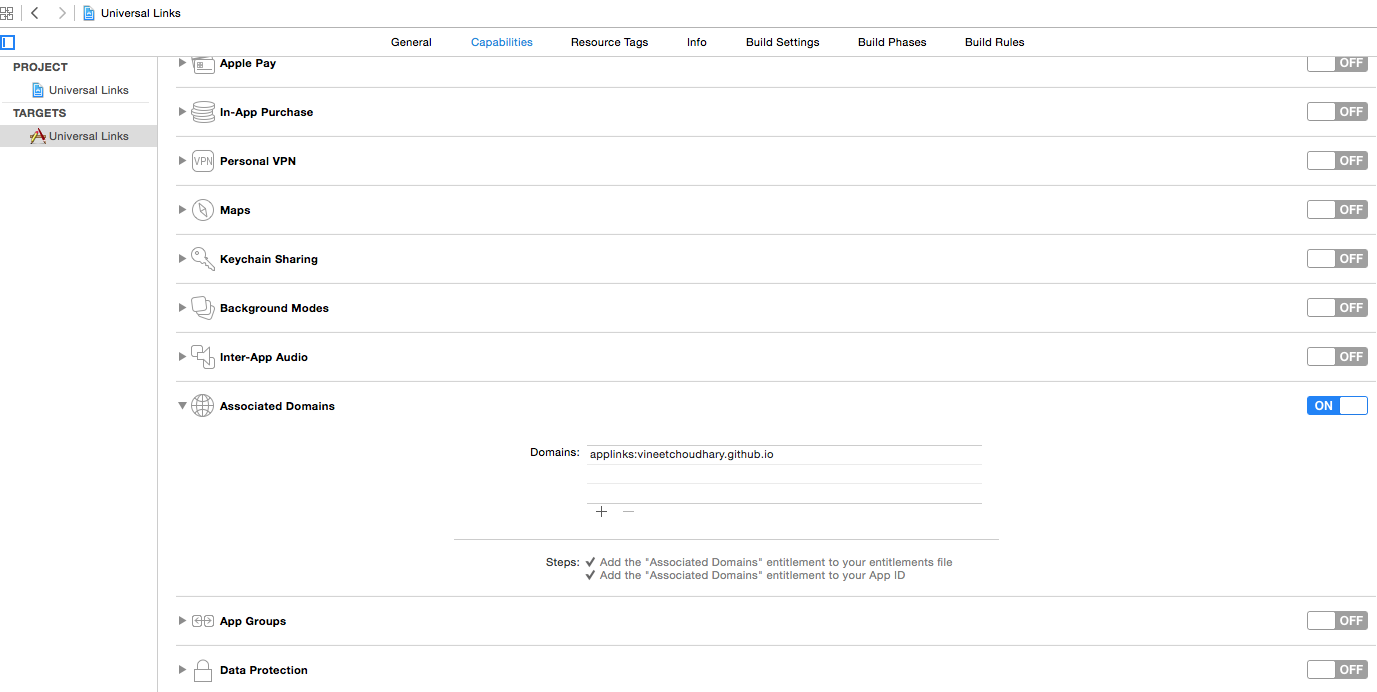
Note: Ensure you have selected the same team and entered the same Bundle ID as the registered App ID on the Member Center. Also ensure that the entitlements file is included by Xcode by selecting the file and in the File Inspector, ensure that your target is checked.
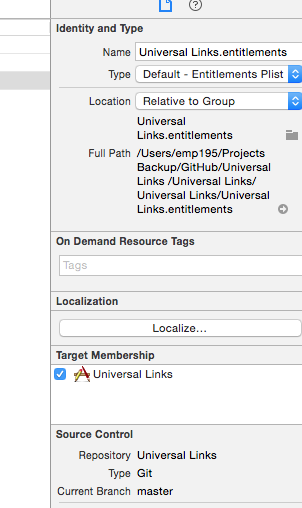
2. Handling Incoming Links in your AppDelegate
All redirects from Safari to the app for universal links go via the below method in the Application’s AppDelegate class. You parse this URL to determine the right action in the app.
[UIApplicationDelegate application: continueUserActivity: restorationHandler:]
Objective-C
-(BOOL)application:(UIApplication *)application continueUserActivity:(NSUserActivity *)userActivity restorationHandler:(void (^)(NSArray * _Nullable))restorationHandler{
///Checking whether the activity was from a web page redirect to the app.
if ([userActivity.activityType isEqualToString: NSUserActivityTypeBrowsingWeb]) {
///Getting the URL from the UserActivty Object.
NSURL *url = userActivity.webpageURL;
UIStoryboard *storyBoard = [UIStoryboard storyboardWithName:@"Main" bundle:nil];
UINavigationController *navigationController = (UINavigationController *)_window.rootViewController;
if ([url.pathComponents containsObject:@"home"]) {
[navigationController pushViewController:[storyBoard instantiateViewControllerWithIdentifier:@"HomeScreenId"] animated:YES];
}else if ([url.pathComponents containsObject:@"about"]){
[navigationController pushViewController:[storyBoard instantiateViewControllerWithIdentifier:@"AboutScreenId"] animated:YES];
}
}
return YES;
}
Swift :
func application(application: UIApplication, continueUserActivity userActivity: NSUserActivity, restorationHandler: ([AnyObject]?) -> Void) -> Bool {
if userActivity.activityType == NSUserActivityTypeBrowsingWeb {
let url = userActivity.webpageURL!
//handle url
}
return true
}
iOS Application Code
The app code can be found master branch here.