UIDynamicBehavior Driven Custom Transition
suggest change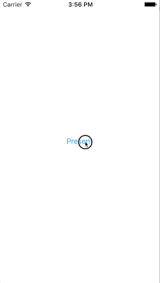
This example shows how to create a custom presentation transition that is driven by a composite UIDynamicBehavior
. We can start by creating a presenting view controller that will present a modal.
Swift
class PresentingViewController: UIViewController
{
lazy var button: UIButton =
{
let button = UIButton()
button.translatesAutoresizingMaskIntoConstraints = false
self.view.addSubview(button)
button.centerXAnchor.constraint(equalTo: self.view.centerXAnchor).isActive
= true
button.centerYAnchor.constraint(equalTo: self.view.centerYAnchor).isActive = true
button.setTitle("Present", for: .normal)
button.setTextColor(UIColor.blue, for: .normal)
return button
}()
override func viewDidLoad()
{
super.viewDidLoad()
button.addTarget(self, action: #selector(self.didPressPresent), for: .touchUpInside)
}
func didPressPresent()
{
let modal = ModalViewController()
modal.view.frame = CGRect(x: 0.0, y: 0.0, width: 200.0, height: 200.0)
modal.modalPresentationStyle = .custom
modal.transitioningDelegate = modal
self.present(modal, animated: true)
}
}
Objective-C
@interface PresentingViewController ()
@property (nonatomic, strong) UIButton *button;
@end
@implementation PresentingViewController
- (void)viewDidLoad
{
[super viewDidLoad];
[self.button addTarget:self action:@selector(didPressPresent) forControlEvents:UIControlEventTouchUpInside];
}
- (void)didPressPresent
{
ModalViewController *modal = [[ModalViewController alloc] init];
modal.view.frame = CGRectMake(0.0, 0.0, 200.0, 200.0);
modal.modalPresentationStyle = UIModalPresentationCustom;
modal.transitioningDelegate = modal;
[self presentViewController:modal animated:YES completion:nil];
}
- (UIButton *)button
{
if (!_button)
{
_button = [[UIButton alloc] init];
_button.translatesAutoresizingMaskIntoConstraints = NO;
[self.view addSubview:_button];
[_button.centerXAnchor constraintEqualToAnchor:self.view.centerXAnchor].active = YES;
[_button.centerYAnchor constraintEqualToAnchor:self.view.centerYAnchor].active = YES;
[_button setTitle:@"Present" forState:UIControlStateNormal];
[_button setTitleColor:[UIColor blueColor] forState:UIControlStateNormal];
}
return _button;
}
@end
When the present button is tapped, we create a ModalViewController
and set its presentation style to .custom
and set its transitionDelegate
to itself. This will allow us to vend an animator that will drive its modal transition. We also set modal
‘s view’s frame so it will be smaller than the full screen.
Let’s now look at ModalViewController
:
Swift
class ModalViewController: UIViewController
{
lazy var button: UIButton =
{
let button = UIButton()
button.translatesAutoresizingMaskIntoConstraints = false
self.view.addSubview(button)
button.centerXAnchor.constraint(equalTo: self.view.centerXAnchor).isActive
= true
button.centerYAnchor.constraint(equalTo: self.view.centerYAnchor).isActive = true
button.setTitle("Dismiss", for: .normal)
button.setTitleColor(.white, for: .normal)
return button
}()
override func viewDidLoad()
{
super.viewDidLoad()
button.addTarget(self, action: #selector(self.didPressDismiss), for: .touchUpInside)
view.backgroundColor = .red
view.layer.cornerRadius = 15.0
}
func didPressDismiss()
{
dismiss(animated: true)
}
}
extension ModalViewController: UIViewControllerTransitioningDelegate
{
func animationController(forPresented presented: UIViewController, presenting: UIViewController, source: UIViewController) -> UIViewControllerAnimatedTransitioning?
{
return DropOutAnimator(duration: 1.5, isAppearing: true)
}
func animationController(forDismissed dismissed: UIViewController) -> UIViewControllerAnimatedTransitioning?
{
return DropOutAnimator(duration: 4.0, isAppearing: false)
}
}
Objective-C
@interface ModalViewController () <UIViewControllerTransitioningDelegate>
@property (nonatomic, strong) UIButton *button;
@end
@implementation ModalViewController
- (void)viewDidLoad
{
[super viewDidLoad];
[self.button addTarget:self action:@selector(didPressPresent) forControlEvents:UIControlEventTouchUpInside];
self.view.backgroundColor = [UIColor redColor];
self.view.layer.cornerRadius = 15.0f;
}
- (void)didPressPresent
{
[self dismissViewControllerAnimated:YES completion:nil];
}
- (UIButton *)button
{
if (!_button)
{
_button = [[UIButton alloc] init];
_button.translatesAutoresizingMaskIntoConstraints = NO;
[self.view addSubview:_button];
[_button.centerXAnchor constraintEqualToAnchor:self.view.centerXAnchor].active = YES;
[_button.centerYAnchor constraintEqualToAnchor:self.view.centerYAnchor].active = YES;
[_button setTitle:@"Dismiss" forState:UIControlStateNormal];
[_button setTitleColor:[UIColor blueColor] forState:UIControlStateNormal];
}
return _button;
}
- (id<UIViewControllerAnimatedTransitioning>)animationControllerForPresentedController:(UIViewController *)presented presentingController:(UIViewController *)presenting sourceController:(UIViewController *)source
{
return [[DropOutAnimator alloc]initWithDuration: 1.5 appearing:YES];
}
- (id<UIViewControllerAnimatedTransitioning>)animationControllerForDismissedController:(UIViewController *)dismissed
{
return [[DropOutAnimator alloc] initWithDuration:4.0 appearing:NO];
}
@end
Here we create the view controller that is presented. Also because ModalViewController
is it’s own transitioningDelegate
it is also responsible for vending an object that will manage its transition animation. For us that means passing on an instance of our composite UIDynamicBehavior
subclass.
Our animator will have two different transitions: one for presenting and one for dismissing. For presenting, the presenting view controller’s view will drop in from above. And for dismissing, the view will seem to swing from a rope and then drop out. Because DropOutAnimator
conforms to UIViewControllerAnimatedTransitioning
most of this work will be done in its implementation of func animateTransition(using transitionContext: UIViewControllerContextTransitioning)
.
Swift
class DropOutAnimator: UIDynamicBehavior
{
let duration: TimeInterval
let isAppearing: Bool
var transitionContext: UIViewControllerContextTransitioning?
var hasElapsedTimeExceededDuration = false
var finishTime: TimeInterval = 0.0
var collisionBehavior: UICollisionBehavior?
var attachmentBehavior: UIAttachmentBehavior?
var animator: UIDynamicAnimator?
init(duration: TimeInterval = 1.0, isAppearing: Bool)
{
self.duration = duration
self.isAppearing = isAppearing
super.init()
}
}
extension DropOutAnimator: UIViewControllerAnimatedTransitioning
{
func animateTransition(using transitionContext: UIViewControllerContextTransitioning)
{
// Get relevant views and view controllers from transitionContext
guard let fromVC = transitionContext.viewController(forKey: .from),
let toVC = transitionContext.viewController(forKey: .to),
let fromView = fromVC.view,
let toView = toVC.view else { return }
let containerView = transitionContext.containerView
let duration = self.transitionDuration(using: transitionContext)
// Hold refrence to transitionContext to notify it of completion
self.transitionContext = transitionContext
// Create dynamic animator
let animator = UIDynamicAnimator(referenceView: containerView)
animator.delegate = self
self.animator = animator
// Presenting Animation
if self.isAppearing
{
fromView.isUserInteractionEnabled = false
// Position toView just off-screen
let fromViewInitialFrame = transitionContext.initialFrame(for: fromVC)
var toViewInitialFrame = toView.frame
toViewInitialFrame.origin.y -= toViewInitialFrame.height
toViewInitialFrame.origin.x = fromViewInitialFrame.width * 0.5 - toViewInitialFrame.width * 0.5
toView.frame = toViewInitialFrame
containerView.addSubview(toView)
// Prevent rotation and adjust bounce
let bodyBehavior = UIDynamicItemBehavior(items: [toView])
bodyBehavior.elasticity = 0.7
bodyBehavior.allowsRotation = false
// Add gravity at exaggerated magnitude so animation doesn't seem slow
let gravityBehavior = UIGravityBehavior(items: [toView])
gravityBehavior.magnitude = 10.0
// Set collision bounds to include off-screen view and have collision in center
// where our final view should come to rest
let collisionBehavior = UICollisionBehavior(items: [toView])
let insets = UIEdgeInsets(top: toViewInitialFrame.minY, left: 0.0, bottom: fromViewInitialFrame.height * 0.5 - toViewInitialFrame.height * 0.5, right: 0.0)
collisionBehavior.setTranslatesReferenceBoundsIntoBoundary(with: insets)
self.collisionBehavior = collisionBehavior
// Keep track of finish time in case we need to end the animator befor the animator pauses
self.finishTime = duration + (self.animator?.elapsedTime ?? 0.0)
// Closure that is called after every "tick" of the animator
// Check if we exceed duration
self.action =
{ [weak self] in
guard let strongSelf = self,
(strongSelf.animator?.elapsedTime ?? 0.0) >= strongSelf.finishTime else { return }
strongSelf.hasElapsedTimeExceededDuration = true
strongSelf.animator?.removeBehavior(strongSelf)
}
// `DropOutAnimator` is a composit behavior, so add child behaviors to self
self.addChildBehavior(collisionBehavior)
self.addChildBehavior(bodyBehavior)
self.addChildBehavior(gravityBehavior)
// Add self to dynamic animator
self.animator?.addBehavior(self)
}
// Dismissing Animation
else
{
// Create allow rotation and have a elastic item
let bodyBehavior = UIDynamicItemBehavior(items: [fromView])
bodyBehavior.elasticity = 0.8
bodyBehavior.angularResistance = 5.0
bodyBehavior.allowsRotation = true
// Create gravity with exaggerated magnitude
let gravityBehavior = UIGravityBehavior(items: [fromView])
gravityBehavior.magnitude = 10.0
// Collision boundary is set to have a floor just below the bottom of the screen
let collisionBehavior = UICollisionBehavior(items: [fromView])
let insets = UIEdgeInsets(top: 0.0, left: -1000, bottom: -225, right: -1000)
collisionBehavior.setTranslatesReferenceBoundsIntoBoundary(with: insets)
self.collisionBehavior = collisionBehavior
// Attachment behavior so view will have effect of hanging from a rope
let offset = UIOffset(horizontal: 70.0, vertical: fromView.bounds.height * 0.5)
var anchorPoint = CGPoint(x: fromView.bounds.maxX - 40.0, y: fromView.bounds.minY)
anchorPoint = containerView.convert(anchorPoint, from: fromView)
let attachmentBehavior = UIAttachmentBehavior(item: fromView, offsetFromCenter: offset, attachedToAnchor: anchorPoint)
attachmentBehavior.frequency = 3.0
attachmentBehavior.damping = 3.0
self.attachmentBehavior = attachmentBehavior
// `DropOutAnimator` is a composit behavior, so add child behaviors to self
self.addChildBehavior(collisionBehavior)
self.addChildBehavior(bodyBehavior)
self.addChildBehavior(gravityBehavior)
self.addChildBehavior(attachmentBehavior)
// Add self to dynamic animator
self.animator?.addBehavior(self)
// Animation has two parts part one is hanging from rope.
// Part two is bouncying off-screen
// Divide duration in two
self.finishTime = (2.0 / 3.0) * duration + (self.animator?.elapsedTime ?? 0.0)
// After every "tick" of animator check if past time limit
self.action =
{ [weak self] in
guard let strongSelf = self,
(strongSelf.animator?.elapsedTime ?? 0.0) >= strongSelf.finishTime else { return }
strongSelf.hasElapsedTimeExceededDuration = true
strongSelf.animator?.removeBehavior(strongSelf)
}
}
}
func transitionDuration(using transitionContext: UIViewControllerContextTransitioning?) -> TimeInterval
{
// Return the duration of the animation
return self.duration
}
}
extension DropOutAnimator: UIDynamicAnimatorDelegate
{
func dynamicAnimatorDidPause(_ animator: UIDynamicAnimator)
{
// Animator has reached stasis
if self.isAppearing
{
// Check if we are out of time
if self.hasElapsedTimeExceededDuration
{
// Move to final positions
let toView = self.transitionContext?.viewController(forKey: .to)?.view
let containerView = self.transitionContext?.containerView
toView?.center = containerView?.center ?? .zero
self.hasElapsedTimeExceededDuration = false
}
// Clean up and call completion
self.transitionContext?.completeTransition(!(self.transitionContext?.transitionWasCancelled ?? false))
self.childBehaviors.forEach { self.removeChildBehavior($0) }
animator.removeAllBehaviors()
self.transitionContext = nil
}
else
{
if let attachmentBehavior = self.attachmentBehavior
{
// If we have an attachment, we are at the end of part one and start part two.
self.removeChildBehavior(attachmentBehavior)
self.attachmentBehavior = nil
animator.addBehavior(self)
let duration = self.transitionDuration(using: self.transitionContext)
self.finishTime = 1.0 / 3.0 * duration + animator.elapsedTime
}
else
{
// Clean up and call completion
let fromView = self.transitionContext?.viewController(forKey: .from)?.view
let toView = self.transitionContext?.viewController(forKey: .to)?.view
fromView?.removeFromSuperview()
toView?.isUserInteractionEnabled = true
self.transitionContext?.completeTransition(!(self.transitionContext?.transitionWasCancelled ?? false))
self.childBehaviors.forEach { self.removeChildBehavior($0) }
animator.removeAllBehaviors()
self.transitionContext = nil
}
}
}
}
Objective-C
@interface ObjcDropOutAnimator() <UIDynamicAnimatorDelegate, UIViewControllerAnimatedTransitioning>
@property (nonatomic, strong) id<UIViewControllerContextTransitioning> transitionContext;
@property (nonatomic, strong) UIDynamicAnimator *animator;
@property (nonatomic, assign) NSTimeInterval finishTime;
@property (nonatomic, assign) BOOL elapsedTimeExceededDuration;
@property (nonatomic, assign, getter=isAppearing) BOOL appearing;
@property (nonatomic, assign) NSTimeInterval duration;
@property (nonatomic, strong) UIAttachmentBehavior *attachBehavior;
@property (nonatomic, strong) UICollisionBehavior * collisionBehavior;
@end
@implementation ObjcDropOutAnimator
- (instancetype)initWithDuration:(NSTimeInterval)duration appearing:(BOOL)appearing
{
self = [super init];
if (self)
{
_duration = duration;
_appearing = appearing;
}
return self;
}
- (void) animateTransition:(id<UIViewControllerContextTransitioning>)transitionContext
{
// Get relevant views and view controllers from transitionContext
UIViewController *fromVC = [transitionContext viewControllerForKey:UITransitionContextFromViewControllerKey];
UIViewController *toVC = [transitionContext viewControllerForKey:UITransitionContextToViewControllerKey];
UIView *fromView = fromVC.view;
UIView *toView = toVC.view;
UIView *containerView = transitionContext.containerView;
NSTimeInterval duration = [self transitionDuration:transitionContext];
// Hold refrence to transitionContext to notify it of completion
self.transitionContext = transitionContext;
// Create dynamic animator
UIDynamicAnimator *animator = [[UIDynamicAnimator alloc]initWithReferenceView:containerView];
animator.delegate = self;
self.animator = animator;
// Presenting Animation
if (self.isAppearing)
{
fromView.userInteractionEnabled = NO;
// Position toView just above screen
CGRect fromViewInitialFrame = [transitionContext initialFrameForViewController:fromVC];
CGRect toViewInitialFrame = toView.frame;
toViewInitialFrame.origin.y -= CGRectGetHeight(toViewInitialFrame);
toViewInitialFrame.origin.x = CGRectGetWidth(fromViewInitialFrame) * 0.5 - CGRectGetWidth(toViewInitialFrame) * 0.5;
toView.frame = toViewInitialFrame;
[containerView addSubview:toView];
// Prevent rotation and adjust bounce
UIDynamicItemBehavior *bodyBehavior = [[UIDynamicItemBehavior alloc]initWithItems:@[toView]];
bodyBehavior.elasticity = 0.7;
bodyBehavior.allowsRotation = NO;
// Add gravity at exaggerated magnitude so animation doesn't seem slow
UIGravityBehavior *gravityBehavior = [[UIGravityBehavior alloc]initWithItems:@[toView]];
gravityBehavior.magnitude = 10.0f;
// Set collision bounds to include off-screen view and have collision floor in center
// where our final view should come to rest
UICollisionBehavior *collisionBehavior = [[UICollisionBehavior alloc]initWithItems:@[toView]];
UIEdgeInsets insets = UIEdgeInsetsMake(CGRectGetMinY(toViewInitialFrame), 0.0, CGRectGetHeight(fromViewInitialFrame) * 0.5 - CGRectGetHeight(toViewInitialFrame) * 0.5, 0.0);
[collisionBehavior setTranslatesReferenceBoundsIntoBoundaryWithInsets:insets];
self.collisionBehavior = collisionBehavior;
// Keep track of finish time in case we need to end the animator befor the animator pauses
self.finishTime = duration + self.animator.elapsedTime;
// Closure that is called after every "tick" of the animator
// Check if we exceed duration
__weak ObjcDropOutAnimator *weakSelf = self;
self.action = ^{
__strong ObjcDropOutAnimator *strongSelf = weakSelf;
if (strongSelf)
{
if (strongSelf.animator.elapsedTime >= strongSelf.finishTime)
{
strongSelf.elapsedTimeExceededDuration = YES;
[strongSelf.animator removeBehavior:strongSelf];
}
}
};
// `DropOutAnimator` is a composit behavior, so add child behaviors to self
[self addChildBehavior:collisionBehavior];
[self addChildBehavior:bodyBehavior];
[self addChildBehavior:gravityBehavior];
// Add self to dynamic animator
[self.animator addBehavior:self];
}
// Dismissing Animation
else
{
// Allow rotation and have a elastic item
UIDynamicItemBehavior *bodyBehavior = [[UIDynamicItemBehavior alloc] initWithItems:@[fromView]];
bodyBehavior.elasticity = 0.8;
bodyBehavior.angularResistance = 5.0;
bodyBehavior.allowsRotation = YES;
// Create gravity with exaggerated magnitude
UIGravityBehavior *gravityBehavior = [[UIGravityBehavior alloc] initWithItems:@[fromView]];
gravityBehavior.magnitude = 10.0f;
// Collision boundary is set to have a floor just below the bottom of the screen
UICollisionBehavior *collisionBehavior = [[UICollisionBehavior alloc] initWithItems:@[fromView]];
UIEdgeInsets insets = UIEdgeInsetsMake(0, -1000, -225, -1000);
[collisionBehavior setTranslatesReferenceBoundsIntoBoundaryWithInsets:insets];
self.collisionBehavior = collisionBehavior;
// Attachment behavior so view will have effect of hanging from a rope
UIOffset offset = UIOffsetMake(70, -(CGRectGetHeight(fromView.bounds) / 2.0));
CGPoint anchorPoint = CGPointMake(CGRectGetMaxX(fromView.bounds) - 40,
CGRectGetMinY(fromView.bounds));
anchorPoint = [containerView convertPoint:anchorPoint fromView:fromView];
UIAttachmentBehavior *attachBehavior = [[UIAttachmentBehavior alloc] initWithItem:fromView offsetFromCenter:offset attachedToAnchor:anchorPoint];
attachBehavior.frequency = 3.0;
attachBehavior.damping = 0.3;
attachBehavior.length = 40;
self.attachBehavior = attachBehavior;
// `DropOutAnimator` is a composit behavior, so add child behaviors to self
[self addChildBehavior:collisionBehavior];
[self addChildBehavior:bodyBehavior];
[self addChildBehavior:gravityBehavior];
[self addChildBehavior:attachBehavior];
// Add self to dynamic animator
[self.animator addBehavior:self];
// Animation has two parts part one is hanging from rope.
// Part two is bouncying off-screen
// Divide duration in two
self.finishTime = (2./3.) * duration + [self.animator elapsedTime];
// After every "tick" of animator check if past time limit
__weak ObjcDropOutAnimator *weakSelf = self;
self.action = ^{
__strong ObjcDropOutAnimator *strongSelf = weakSelf;
if (strongSelf)
{
if ([strongSelf.animator elapsedTime] >= strongSelf.finishTime)
{
strongSelf.elapsedTimeExceededDuration = YES;
[strongSelf.animator removeBehavior:strongSelf];
}
}
};
}
}
- (NSTimeInterval)transitionDuration:(id<UIViewControllerContextTransitioning>)transitionContext
{
return self.duration;
}
- (void)dynamicAnimatorDidPause:(UIDynamicAnimator *)animator
{
// Animator has reached stasis
if (self.isAppearing)
{
// Check if we are out of time
if (self.elapsedTimeExceededDuration)
{
// Move to final positions
UIView *toView = [self.transitionContext viewControllerForKey:UITransitionContextToViewControllerKey].view;
UIView *containerView = [self.transitionContext containerView];
toView.center = containerView.center;
self.elapsedTimeExceededDuration = NO;
}
// Clean up and call completion
[self.transitionContext completeTransition:![self.transitionContext transitionWasCancelled]];
for (UIDynamicBehavior *behavior in self.childBehaviors)
{
[self removeChildBehavior:behavior];
}
[animator removeAllBehaviors];
self.transitionContext = nil;
}
// Dismissing
else
{
if (self.attachBehavior)
{
// If we have an attachment, we are at the end of part one and start part two.
[self removeChildBehavior:self.attachBehavior];
self.attachBehavior = nil;
[animator addBehavior:self];
NSTimeInterval duration = [self transitionDuration:self.transitionContext];
self.finishTime = 1./3. * duration + [animator elapsedTime];
}
else
{
// Clean up and call completion
UIView *fromView = [self.transitionContext viewControllerForKey:UITransitionContextFromViewControllerKey].view;
UIView *toView = [self.transitionContext viewControllerForKey:UITransitionContextToViewControllerKey].view;
[fromView removeFromSuperview];
toView.userInteractionEnabled = YES;
[self.transitionContext completeTransition:![self.transitionContext transitionWasCancelled]];
for (UIDynamicBehavior *behavior in self.childBehaviors)
{
[self removeChildBehavior:behavior];
}
[animator removeAllBehaviors];
self.transitionContext = nil;
}
}
}
As composite behavior, DropOutAnimator
, can combine a number of different behaviors to perform its presenting and dismissing animations. DropOutAnimator
also demonstrates how to use the action
block of a behavior to inspect the locations of its items as well as the time elapsed a technique that can be used to remove views that move offscreen or truncate animations that have yet to reach stasis.
For more information 2013 WWDC Session “Advanced Techniques with UIKit Dynamics” as well as SOLPresentingFun