Random with gaussian distribution
suggest changeThe Math.random()
function should give random numbers that have a standard deviation approaching 0. When picking from a deck of card, or simulating a dice roll this is what we want.
But in most situations this is unrealistic. In the real world the randomness tends to gather around an common normal value. If plotted on a graph you get the classical bell curve or gaussian distribution.
To do this with the Math.random()
function is relatively simple.
var randNum = (Math.random() + Math.random()) / 2;
var randNum = (Math.random() + Math.random() + Math.random()) / 3;
var randNum = (Math.random() + Math.random() + Math.random() + Math.random()) / 4;
Adding a random value to the last increases the variance of the random numbers. Dividing by the number of times you add normalises the result to a range of 0–1
As adding more than a few randoms is messy a simple function will allow you to select a variance you want.
// v is the number of times random is summed and should be over >= 1
// return a random number between 0-1 exclusive
function randomG(v){
var r = 0;
for(var i = v; i > 0; i --){
r += Math.random();
}
return r / v;
}
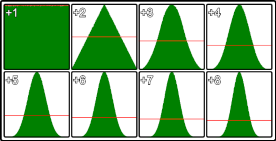
The image shows the distribution of random values for different values of v. The top left is standard single Math.random()
call the bottom right is Math.random()
summed 8 times. This is from 5,000,000 samples using Chrome
This method is most efficient at v < 5
.